1. In my project create an "Entity from table" using the new object gallery item in the EJB node. In this example we use the classic DEPT table.
2. Then edit your Dept entity to create another named query as shown below, as well as a toString method to display the bean.
....
@Entity
@NamedQueries({
@NamedQuery(name = "Dept.findAll", query = "select o from Dept o"),
@NamedQuery(name = "Dept.findByDeptno", query = "select o from Dept o where o.deptno = :deptno")
})
public class Dept
implements Serializable
...
@Override
public String toString()
{
return "Dept [" + deptno + ", " + dname + ", " + loc + "]";
}
3. From the EJB new object gallery select "Java Service Facade", in the wizard I only exposed these methods as well as a main method to test it with.
- public List
getDeptFindAll() public - public List
getDeptFindByDeptno(Long deptno)
public static void main(String [] args)
{
final JavaServiceFacade javaServiceFacade = new JavaServiceFacade();
// TODO: Call methods on javaServiceFacade here...
List<Dept> deps = javaServiceFacade.getDeptFindAll();
for (Dept d: deps)
{
System.out.println(d);
}
}
5. Run it to verify it works well. Inside the persistence.xml I edited the following property (eclipselink.logging.level=INFO) to reduce the amount of output we get at runtime.
<persistence-unit name="Model-Test" transaction-type="RESOURCE_LOCAL">
<provider>org.eclipse.persistence.jpa.PersistenceProvider</provider>
<class>model.Dept</class>
<properties>
<property name="eclipselink.jdbc.driver"
value="oracle.jdbc.OracleDriver"/>
<property name="eclipselink.jdbc.url"
value="jdbc:oracle:thin:@//beast.au.oracle.com:1523/linux11gr2"/>
<property name="eclipselink.jdbc.user" value="scott"/>
<property name="eclipselink.jdbc.password"
value="3E20F8982C53F4ABA825E30206EC8ADE"/>
<property name="eclipselink.logging.level" value="INFO"/>
<property name="eclipselink.target-server" value="WebLogic_10"/>
</properties>
</persistence-unit>
Output:
... [EL Warning]: 2009-12-24 11:48:40.203--Failed to get InitialContext for MBean registration: javax.naming.NoInitialContextException: Need to specify class name in environment or system property, or as an applet parameter, or in an application resource file: java.naming.factory.initial
Dept [10, ACCOUNTING, NEW YORK]
Dept [20, RESEARCH, DALLAS]
Dept [30, SALES, CHICAGO]
Dept [40, OPERATIONS, BOSTON]
6. Now finally create a web service from the "JavaServiceFacade.java". To do this I simply invoked the "Create Java Web Service" from the object gallery or even easier use it's context menu option "Create Web Service".
Now you can test it using the "Test Web Service Wizard" option from the web service itself as now it is a Web Service as per step #6.
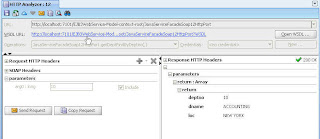
1 comment:
I have been visiting various blogs for my term papers writing research. I have found your blog to be quite useful. Keep updating your blog with valuable information... Regards
Post a Comment